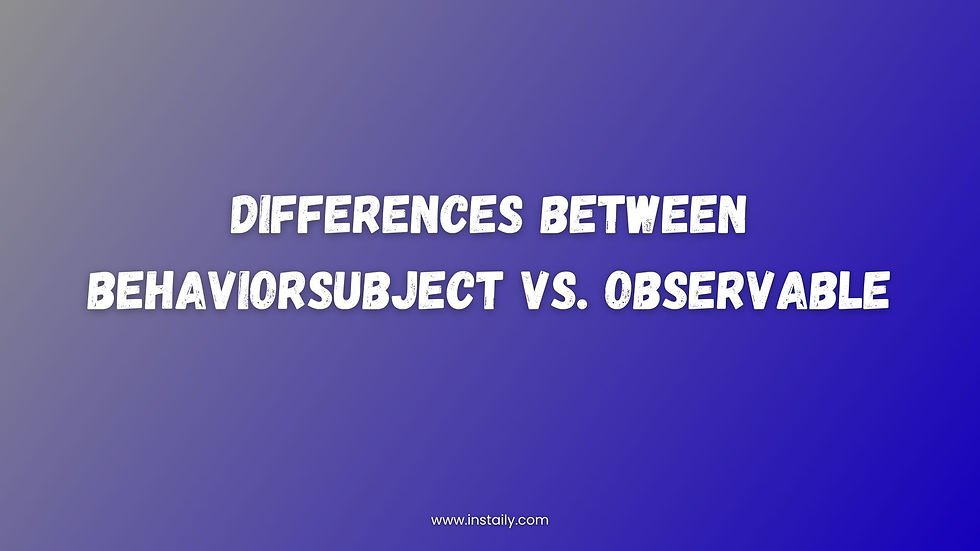
If you are working with Angular, React, or any other framework that uses reactive programming, you may have encountered the terms BehaviorSubject and Observable. These are two of the most common concepts in RxJS, a library that provides tools for working with asynchronous and event-based data streams. In this blog post, we will explain what BehaviorSubject and Observable are, how they differ, and when to use them.
What is Observable?
An Observable is a function that returns values over time when you subscribe to it. You can think of an Observable as a source of data that can emit zero or more values, and optionally a completion or an error signal. For example, an Observable can represent a user input, a timer, a web request, or any other event that occurs asynchronously.
To create an Observable, you can use one of the many creation operators provided by RxJS, such as of, from, interval, ajax, etc. For example, you can create an Observable that emits the numbers 1, 2, and 3, and then completes, like this:
import { of } from 'rxjs';
// Create an Observable that emits 1, 2, and 3
const observable = of(1, 2, 3);
To use an Observable, you need to subscribe to it, which means registering a function that will be called when the Observable emits a value, completes, or errors. For example, you can subscribe to the Observable we created above, and log the values to the console, like this:
// Subscribe to the Observable
observable.subscribe(
// The function to be called when a value is emitted
value => console.log(value),
// The function to be called when an error occurs
error => console.error(error),
// The function to be called when the Observable completes
() => console.log('Completed')
);
// Output:
// 1
// 2
// 3
// Completed
Note that an Observable is lazy, which means that it does not start emitting values until you subscribe to it. Also, an Observable can have multiple subscribers, but each subscriber will receive its own independent execution of the Observable. This means that each subscriber will get the same values in the same order, but at different times.
What is BehaviorSubject?
A BehaviorSubject is a special type of Observable that can emit the current value (Observables have no concept of current value). A BehaviorSubject is also a Subject, which means that it can act as both an Observable and an Observer. As an Observable, it can emit values and be subscribed to. As an Observer, it can receive values and pass them to its subscribers.
To create a BehaviorSubject, you need to provide an initial value that will be emitted when the BehaviorSubject is subscribed to. For example, you can create a BehaviorSubject that has an initial value of 0, like this:
import { BehaviorSubject } from 'rxjs';
// Create a BehaviorSubject with an initial value of 0
const behaviorSubject = new BehaviorSubject(0);
To use a BehaviorSubject, you can subscribe to it, just like an Observable, and receive the current value and any subsequent values. For example, you can subscribe to the BehaviorSubject we created above, and log the values to the console, like this:
// Subscribe to the BehaviorSubject
behaviorSubject.subscribe(
// The function to be called when a value is emitted
value => console.log(value),
// The function to be called when an error occurs
error => console.error(error),
// The function to be called when the BehaviorSubject completes
() => console.log('Completed')
);
// Output:
// 0
Note that a BehaviorSubject will always emit the current value to new subscribers, even if it has not received any new values since the last subscription. Also, a BehaviorSubject can have multiple subscribers, and they will all receive the same values at the same time.
To change the current value of a BehaviorSubject, you can use the next() method, which will emit the new value to all subscribers. For example, you can change the current value of the BehaviorSubject we created above to 1, 2, and 3, like this:
// Change the current value of the BehaviorSubject to 1
behaviorSubject.next(1);
// Change the current value of the BehaviorSubject to 2
behaviorSubject.next(2);
// Change the current value of the BehaviorSubject to 3
behaviorSubject.next(3);
// Output:
// 1
// 2
// 3
Note that a BehaviorSubject can also complete an error, just like an Observable, by using the complete() or error() methods. However, once a BehaviorSubject completes or errors, it will no longer emit any values, and any new subscribers will receive the completion or error signal.
BehaviorSubject vs Observable: Key Differences
Now that we have seen what BehaviorSubject and Observable are, let us summarize the key differences between them:
A BehaviorSubject is a Subject that can emit the current value, while an Observable has no concept of current value.
A BehaviorSubject requires an initial value, while an Observable does not.
A BehaviorSubject emits the current value to new subscribers, while an Observable emits values only when they are generated.
A BehaviorSubject can change its current value by using the next() method, while an Observable cannot.
A BehaviorSubject can have multiple subscribers that receive the same values at the same time, while an Observable can have multiple subscribers that receive the same values in the same order, but at different times.
BehaviorSubject vs Observable: When to Use Them
The choice between BehaviorSubject and Observable depends on the use case and the requirements of the application. Here are some general guidelines on when to use them:
Use an Observable when you want to represent a stream of data that can be emitted over time, and that does not depend on the previous or current values. For example, use an Observable for user input, web requests, timers, etc.
Use a BehaviorSubject when you want to represent a stream of data that has a current value, and that can be changed and shared with other components. For example, use a BehaviorSubject for application state, user preferences, configuration settings, etc.
Conclusion
In this blog post, we have explained what BehaviorSubject and Observable are, how they differ, and when to use them.
Comments