Angular Component 101: A Crash Course
- Instaily
- Sep 8, 2023
- 6 min read
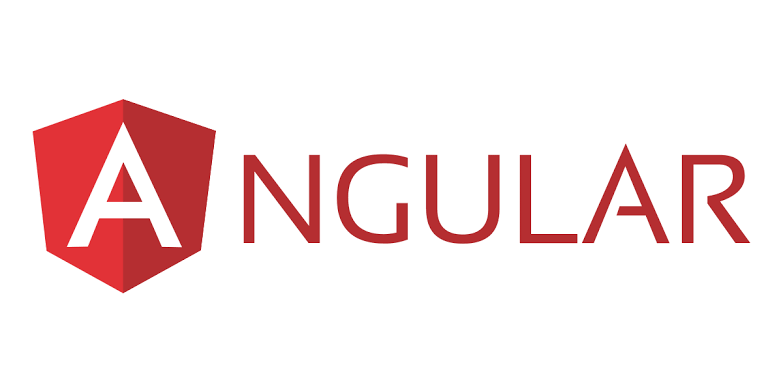
In the ever-evolving landscape of web development, staying ahead of the curve is crucial. Angular- a robust and versatile framework that has taken the world of modern web application development by storm. At the core of Angular's power lies its component-based architecture, a paradigm that empowers developers to create dynamic, modular, and scalable web applications.
Did you know that the demand for Angular skills continues to surge, with organizations actively seeking professionals who can leverage the capabilities of Angular to craft exceptional user experiences? This fact underscores the growing importance of Angular as a skill that can catapult your career to new heights.
In this concise yet comprehensive guide, we embark on a journey through the fundamental building blocks of Angular development, with a primary focus on Angular Component Basics. These components serve as the heart and soul of your web applications, encapsulating both the logic and the visual elements that breathe life into your creations.
But this isn't your average Angular tutorial. This is a crash course—a condensed, hands-on experience designed to equip you with the essential skills and knowledge needed to harness the full potential of Angular components. Whether you're new to Angular or looking to refresh your skills, this guide will empower you to build dynamic and interactive web applications.
Over the next sections, we'll dive into the core concepts of Angular components, provide practical guidance on setting up your development environment, and walk you through the process of creating your very first Angular component. But we won't stop there. We'll explore data binding, component communication, styling, lifecycle hooks, routing, testing, and even touch on advanced concepts. By the end of this journey, you'll be well-versed in Angular development, ready to tackle real-world projects with confidence.
So, fasten your seatbelts and get ready to embark on this exhilarating journey through Angular Component 101. When we're done, you'll possess the knowledge and skills to build web applications that stand out in today's competitive digital landscape.
Stay tuned as we delve into the world of Angular components. Our next destination is a deeper exploration of what Angular components are and why they're the cornerstone of web development in the Angular ecosystem. Let's get started on this exciting adventure.
Understanding Angular Components
Setting Up Your Angular Environment
Before we dive into the world of Angular components, it's essential to ensure you have the right tools and environment set up for a smooth development experience. Angular development typically relies on a few key components:
Node.js: Angular is built on Node.js, a JavaScript runtime environment. It not only enables you to run JavaScript on the server side but also includes npm (Node Package Manager), a crucial tool for managing packages and dependencies in your Angular projects.
Angular CLI: The Angular CLI (Command Line Interface) is a powerful tool that simplifies many aspects of Angular development. It allows you to create projects, generate components, services, and more, all with a few simple commands.
Here's a quick guide on how to set up your development environment:
Install Node.js and npm: Start by downloading and installing Node.js from the official website (https://nodejs.org/). This installation will include npm, which you'll use to manage packages.
Install Angular CLI: Open your command prompt or terminal and run the following command to install Angular CLI globally:
npm install -g @angular/cli
Verify Installation: After installation is complete, you can verify that Node.js, npm, and Angular CLI are properly installed by running the following commands:
node -v
npm -v
ng --version
You should see the version numbers displayed in your terminal.
With your environment now configured, you're ready to start building Angular components.
Creating Your First Angular Component
Angular components are the building blocks of your web application's user interface. They encapsulate the presentation logic and user interface elements, making your code organized and maintainable.
Let's create your first Angular component using the Angular CLI:
Generate a New Component: Open your terminal and navigate to your project's root directory. Run the following command to generate a new component:
ng generate component my-component
Replace my-component with the desired name for your component.
Explore the Generated Files: After running the command, the Angular CLI will create several files and directories for your component, including:
my-component.component.ts: This TypeScript file contains the component's logic and behavior.
my-component.component.html: This HTML file defines the component's template.
my-component.component.css: This CSS file allows you to style your component.
Edit the Component: Open the my-component.component.ts file in your code editor. Here, you can define properties, methods, and other logic specific to this component.
Customize the Template: Modify the my-component.component.html file to create the visual representation of your component. You can use HTML and Angular-specific templates to structure your component's UI.
Style Your Component: If needed, add styles to the my-component.component.css file to control the component's appearance.
Include the Component: To use your new component, you'll need to include it in other parts of your application, such as within other components or in your app's routing configuration.
With your first Angular component created, you've taken your first step into the world of Angular development. Angular's component-based architecture promotes reusability, maintainability, and scalability—key principles in modern web development.
In the next section, we'll explore data binding in Angular components, a fundamental concept that enables your components to interact with and display dynamic data. Get ready to dive into the heart of Angular development.
Data Binding in Angular Components
Understanding Data Binding
One of the cornerstones of modern web development is the ability to create dynamic and interactive user interfaces. Angular simplifies this process with a powerful feature called data binding. At its core, data binding is the automatic synchronization of data between the component and the view, allowing your application to respond to user input and change data dynamically.
Data binding in Angular can be divided into several types:
Interpolation: This is a one-way data binding method that allows you to embed component data into the template. It's as simple as placing a variable within double curly braces {{}} in your HTML template. For example:
<h1>Welcome, {{ username }}!</h1>
Here, the username variable in the component is interpolated into the template.
Property Binding: With property binding, you can set an HTML element's property to a value from the component. It's commonly used for enabling or disabling elements, changing attributes, or setting the source of an image. Here's an example:
<button [disabled]="isDisabled">Click me</button>
In this case, the isDisabled property in the component controls whether the button is disabled or not.
Event Binding: Event binding allows you to listen for events raised by the user, such as clicking a button or typing in an input field, and respond to those events with methods defined in the component. Here's a simple click event binding example:
<button (click)="onClick()">Click me</button>
In this case, the onClick() method in the component is executed when the button is clicked.
Two-way Binding: Two-way binding combines property binding and event binding to achieve two-way communication between the component and the view. It's often used for forms and input elements, ensuring that changes made by the user are reflected in the component and vice versa. Here's an example using [(ngModel)]:
<input [(ngModel)]="username">
This binds the input element's value to the username property in the component.
Data binding is a powerful concept that simplifies the development of interactive web applications. It allows you to create user interfaces that respond to user actions, making your applications more engaging and user-friendly.
In the next section, we'll explore how Angular components can communicate with each other, enabling the building of complex and interconnected web applications. Component communication is a crucial skill for any Angular developer, and we'll dive into it shortly.
Component Communication in Angular
The Importance of Component Communication
In a real-world web application, you often encounter scenarios where different parts of your application need to communicate and share data. Angular, with its component-based architecture, provides powerful mechanisms for achieving this inter-component communication.
Let's understand why component communication is crucial:
Modularity: Angular encourages breaking your application into smaller, reusable components. These components should be able to communicate effectively to maintain modularity.
Complex UIs: In complex user interfaces, various components may need to work together to display data or respond to user interactions.
Parent-Child Relationships: In many cases, you'll have a hierarchy of components, with parent components containing child components. Communication between them is essential for passing data and triggering actions.
Methods of Component Communication
Angular offers several methods for components to communicate with each other:
Input Properties: One-way communication from parent to child components is achieved through input properties. The parent component passes data to the child component through these properties.
Output Properties and Event Emitters: Child components can communicate with parent components by emitting custom events using Output properties and EventEmitter.
Services: Angular services act as intermediaries, facilitating communication between components that may not have a direct parent-child relationship.
@ViewChild and @ContentChild: These decorators allow parent components to access child components directly in the component code.
RxJS Observables: Observable data streams enable complex communication scenarios, including broadcasting events to multiple subscribers.
NgRx Store: For managing application state and enabling communication between various components, especially in large-scale applications.
Each of these methods has its use cases, and choosing the right one depends on the specific requirements of your application.
In the upcoming sections, we'll explore these methods in detail, providing practical examples and scenarios for effective component communication. By the end of this section, you'll have a comprehensive understanding of how to enable seamless interactions between your Angular components.
Stay tuned as we dive into the intricacies of input properties and output properties, laying the foundation for effective parent-child component communication in Angular.
Comments