Mastering React Native Styles: A Comprehensive Guide to Styling Components
- Instaily
- Nov 14, 2023
- 3 min read
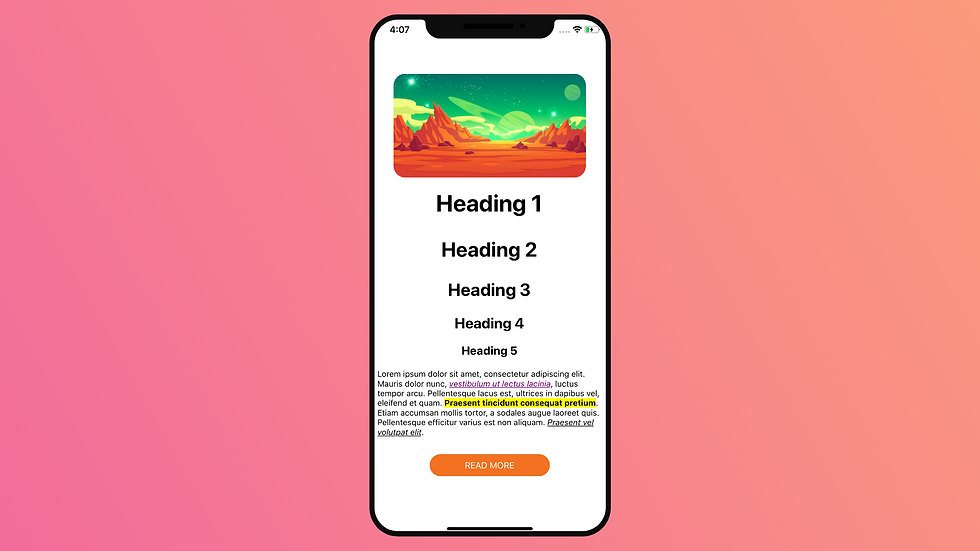
In the dynamic world of React Native development, creating visually appealing and responsive user interfaces is a skill that sets exceptional developers apart. As we delve into the nuances of React Native styles, this comprehensive guide aims to equip you with the knowledge and techniques to master the art of styling components. Whether you're a beginner eager to enhance your UI design skills or an experienced developer looking to deepen your understanding, this guide will cover the fundamental concepts and advanced strategies for styling React Native components.
Understanding the Basics of React Native Styles
Before we embark on the journey of mastering React Native styles, let's establish a solid foundation by exploring the basics.
1. Inline Styles
Similar to React on the web, React Native supports inline styles. You can apply styles directly to components using the style prop. This allows for a straightforward approach to styling individual components.
import React from 'react';
import { View, Text, StyleSheet } from 'react-native';
const MyComponent = () => {
return (
<View style={{ backgroundColor: 'lightblue', padding: 10 }}>
<Text style={{ fontSize: 18, color: 'darkblue' }}>Hello, React Native!</Text>
</View>
);
};
2. External Stylesheets
For a more organized and reusable approach, you can use external stylesheets. Define your styles in a separate file and import them into your components.
// styles.js
import { StyleSheet } from 'react-native';
export const commonStyles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
},
text: {
fontSize: 16,
color: 'black',
},
});
// MyComponent.js
import React from 'react';
import { View, Text } from 'react-native';
import { commonStyles } from './styles';
const MyComponent = () => {
return (
<View style={commonStyles.container}>
<Text style={commonStyles.text}>Styled Component</Text>
</View>
);
};
Advanced Styling Techniques
Now that we've covered the basics, let's explore advanced styling techniques to elevate your React Native UI design.
1. Flexbox Layout
React Native employs Flexbox for layout design, allowing you to create flexible and responsive interfaces. Understanding Flexbox concepts like flexDirection, justifyContent, and alignItems is crucial for building dynamic layouts.
const FlexboxExample = () => {
return (
<View style={{ flex: 1, flexDirection: 'column', justifyContent: 'space-between', alignItems: 'center' }}>
<View style={{ width: 50, height: 50, backgroundColor: 'red' }} />
<View style={{ width: 50, height: 50, backgroundColor: 'green' }} />
<View style={{ width: 50, height: 50, backgroundColor: 'blue' }} />
</View>
);
};
2. Platform-Specific Styling
React Native allows you to customize styles based on the platform, ensuring a consistent look and feel across iOS and Android.
import { Platform, StyleSheet } from 'react-native';
const styles = StyleSheet.create({
container: {
padding: 10,
...Platform.select({
ios: {
backgroundColor: 'lightblue',
},
android: {
backgroundColor: 'lightgreen',
},
}),
},
});
3. Theming with Context API
Implementing a theme for your React Native application becomes more manageable with the Context API. Create a theme context and use it to dynamically apply styles based on the selected theme.
import React, { createContext, useContext } from 'react';
import { View, Text, StyleSheet } from 'react-native';
const ThemeContext = createContext();
const MyThemedComponent = () => {
const theme = useContext(ThemeContext);
const styles = StyleSheet.create({
container: {
backgroundColor: theme.backgroundColor,
padding: 10,
},
text: {
fontSize: 18,
color: theme.textColor,
},
});
return (
<View style={styles.container}>
<Text style={styles.text}>Themed Component</Text>
</View>
);
};
// In a parent component
const App = () => {
const theme = { backgroundColor: 'lightblue', textColor: 'darkblue' };
return (
<ThemeContext.Provider value={theme}>
<MyThemedComponent />
</ThemeContext.Provider>
);
};
Debugging Styles in React Native
Effectively debugging styles is an essential skill for React Native developers. Leverage tools like React DevTools and the built-in StyleSheet API to identify and resolve styling issues efficiently.
// Enable React DevTools in your app
import { AppRegistry } from 'react-native';
import App from './App';
import { name as appName } from './app.json';
if (__DEV__) {
require('react-devtools');
}
AppRegistry.registerComponent(appName, () => App);
Conclusion:
Mastering React Native styles is a journey that combines fundamental principles with creativity. By understanding the basics of inline styles, stylesheets, and Flexbox, you lay the foundation for creating aesthetically pleasing layouts. As you delve into more advanced styling techniques and explore libraries, you'll unlock the full potential of React Native for crafting visually stunning and user-friendly mobile applications.
Embark on this styling adventure, experiment with different techniques, and let your creativity shine as you elevate the design of your React Native components!
Comments